ListView is an important component of android app development so understanding using ListViews is a compulsory skill. In this tutorial, we will learn how to use Simple ListView ArrayAdapter to show items. ListViews are used to display multiple items from arrays. ArrayAdapter works as a bridge between data array and ListViews.
Final Output of the app
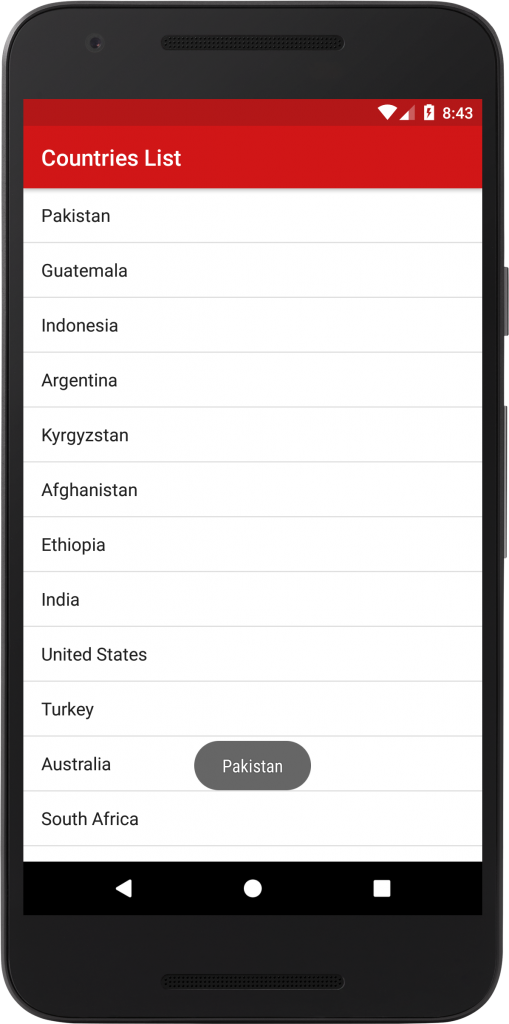
DEMO
1. App name, Package name, and Project location.
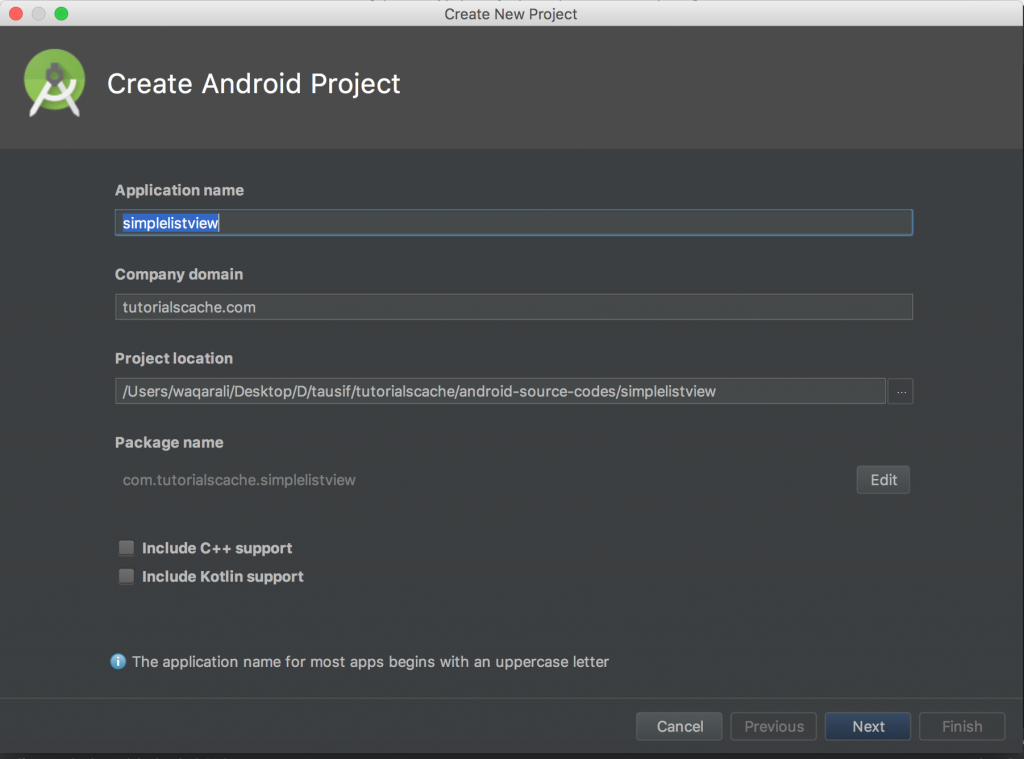
2. Choosing SDK
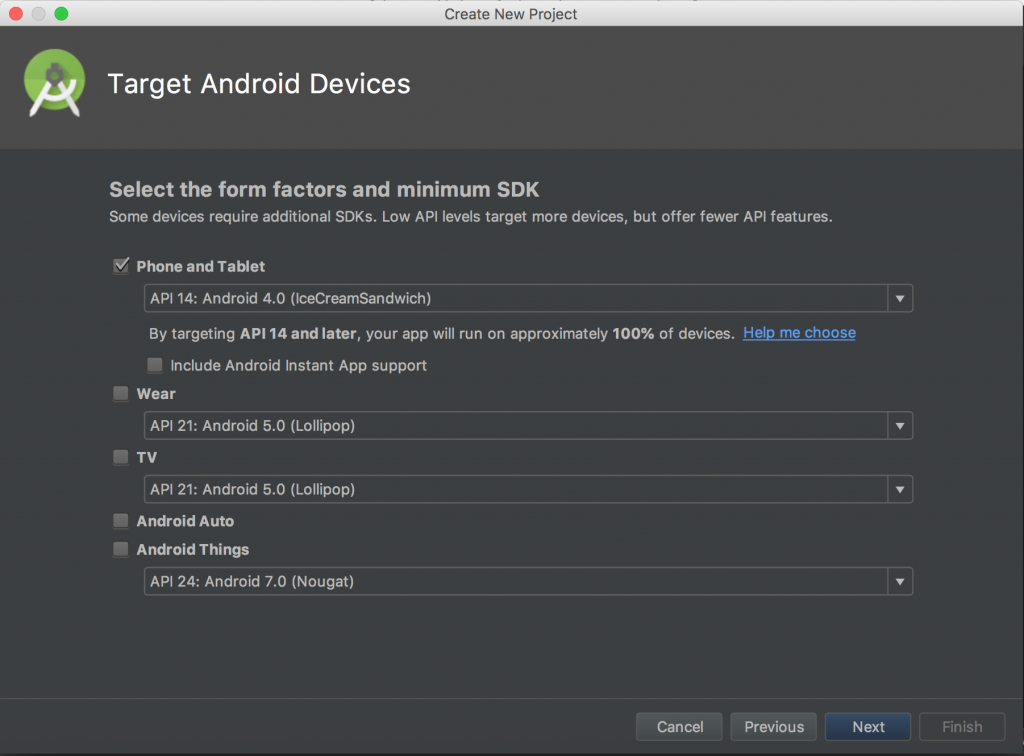
3. Choose Empty Activity
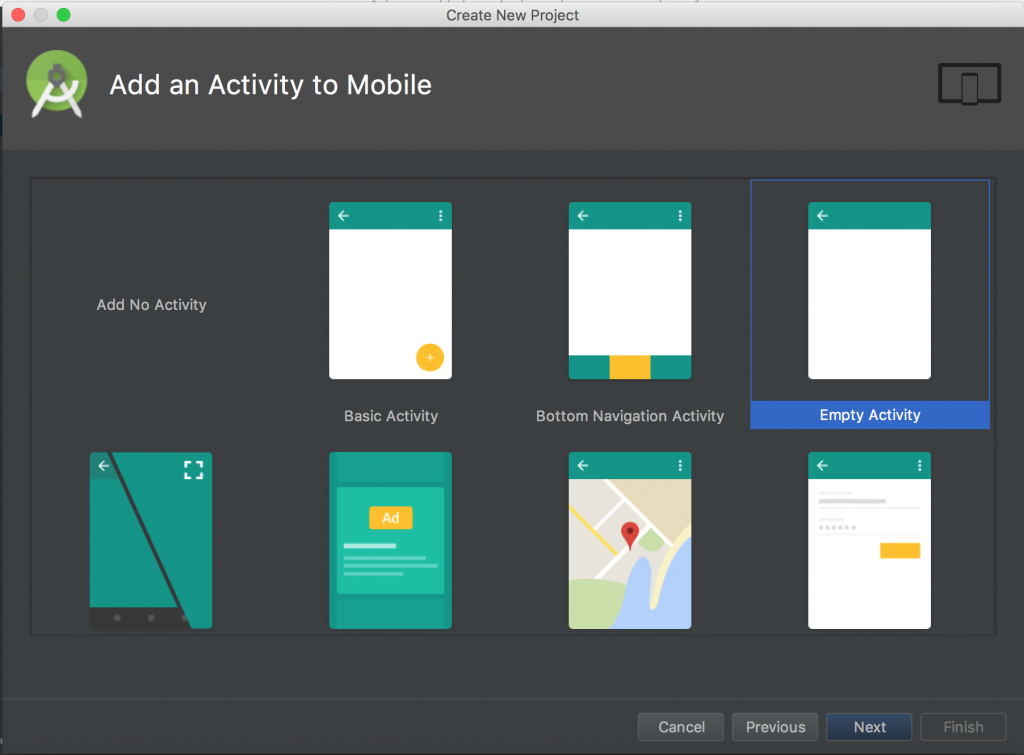
4. Adding code into activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.tutorialscache.simplelistview.MainActivity"> <ListView android:id="@+id/listView" android:background="#ffffff" android:scrollbars="none" android:layout_width="match_parent" android:layout_height="match_parent"/> </LinearLayout>
4. MainActivity.java
package com.tutorialscache.simplelistview; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.AdapterView; import android.widget.ArrayAdapter; import android.widget.ListView; import android.widget.Toast; public class MainActivity extends AppCompatActivity { //array of strings String[] countryNames = {"Pakistan","Guatemala","Indonesia","Argentina","Kyrgyzstan","Afghanistan","Ethiopia","India", "United States","Turkey","Australia","South Africa","Egypt"}; ListView listView; ArrayAdapter<String> arrayAdapter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //connecting listview from activity_main.xml layout listView = findViewById(R.id.listView); //initializing adapter arrayAdapter = new ArrayAdapter<>(getApplicationContext(),android.R.layout.simple_list_item_1,countryNames); //setting ArrayAdapter on listView listView.setAdapter(arrayAdapter); //making listView clickable listView.setOnItemClickListener(new AdapterView.OnItemClickListener() { @Override public void onItemClick(AdapterView<?> adapterView, View view, int position, long l) { String countryName = countryNames[position]; //showing clicked item on toast Toast.makeText(getApplicationContext(),countryName,Toast.LENGTH_LONG).show(); } }); } }
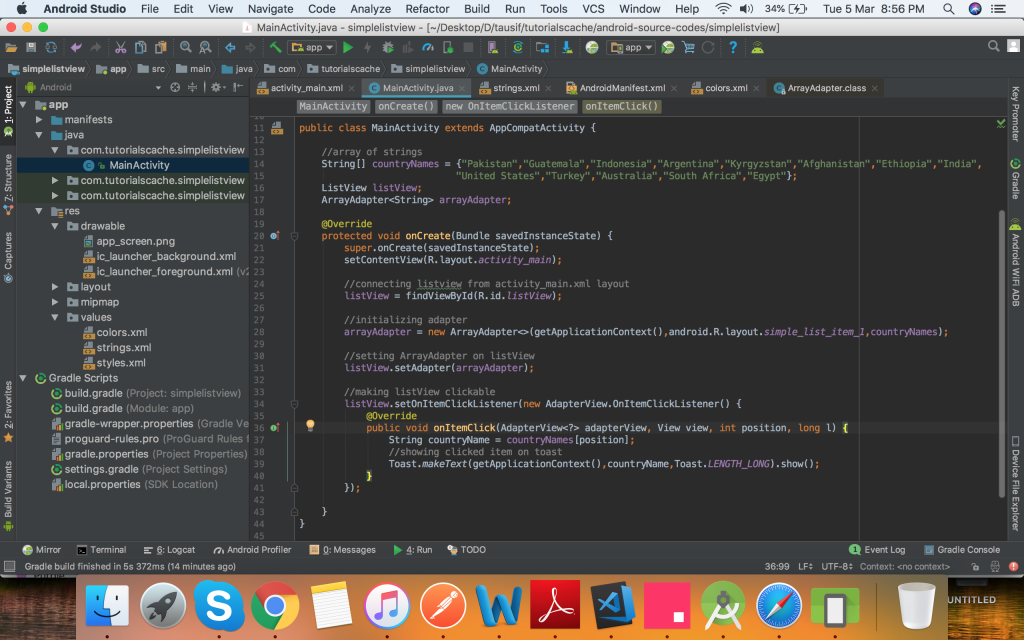
Comments are closed.