Social logins in mobile applications are the quickest way to log in/signup. In this tutorial, we will learn how to implement Facebook login inside an android application using a custom login button. Facebook provides its own login button using Facebook SDK but we need to do that using a custom button so we can use any fancy style button on the login/signup pages of the application.
In order to complete this task first, we need to create our app in the Facebook developers section. Facebook will provide us with tokens and secrets which we will integrate into the application. Here are the steps to follow for the creation of an App under Facebook Developers.
1. Create Facebook App ID
Open https://developers.facebook.com/ click on My Apps > Create App. Enter the App display name and email and press on Create AppID button.
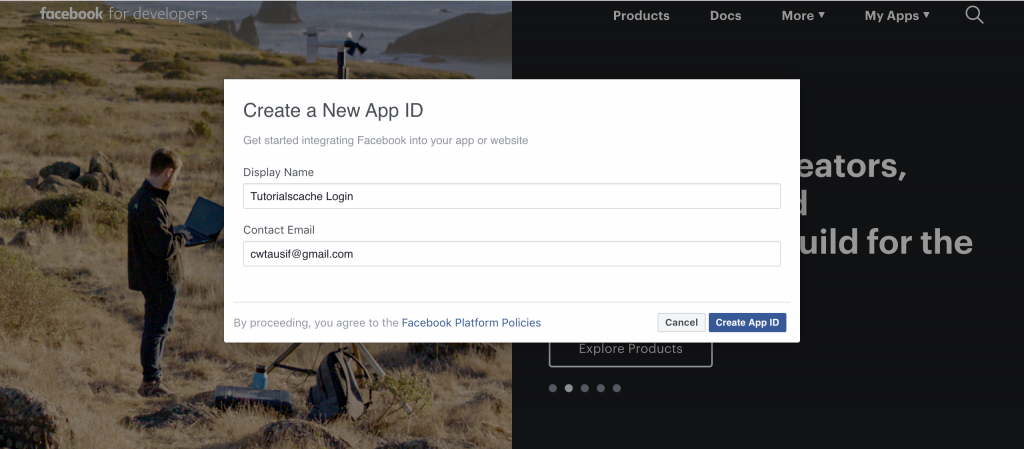
The next step is to choose Integrate Facebook Login option from the dashboard and click confirm.
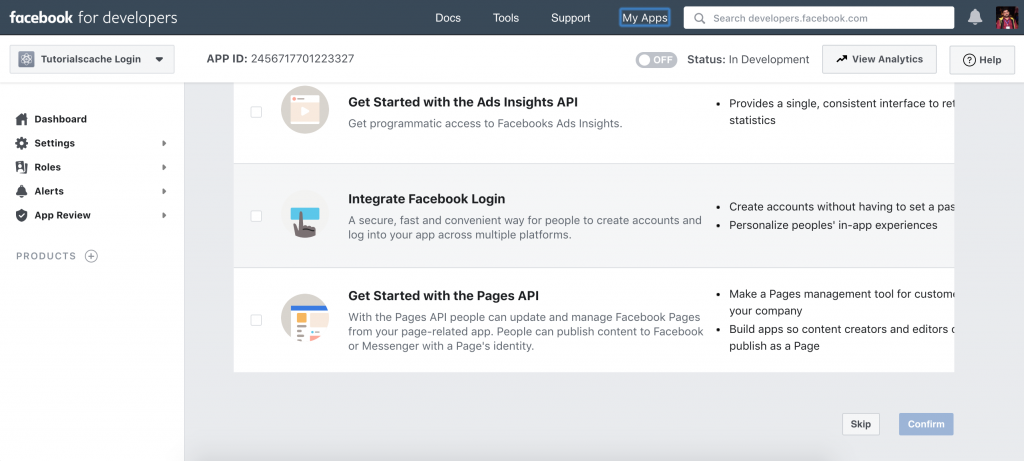
In the following step now we need to choose Android.
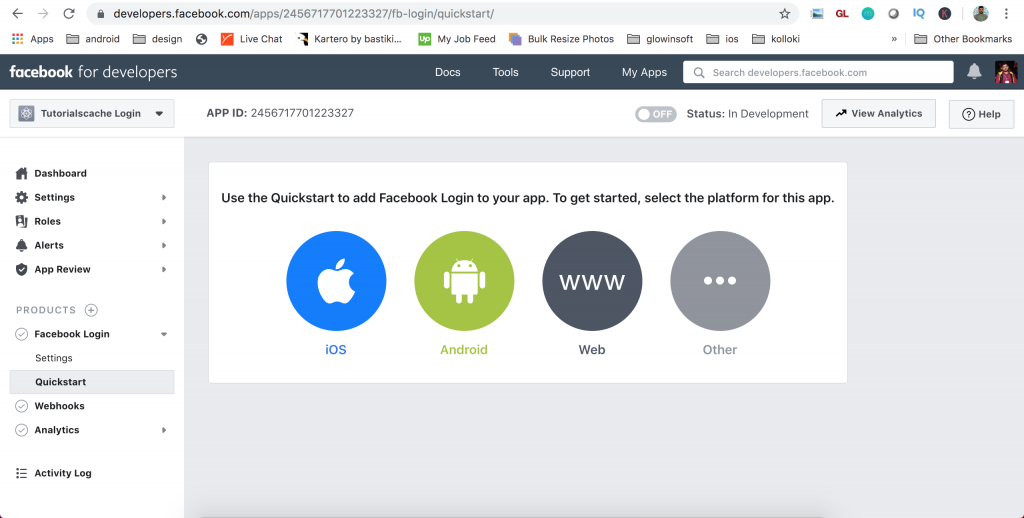
Add mavenCenteral() into build.gradle (Project).
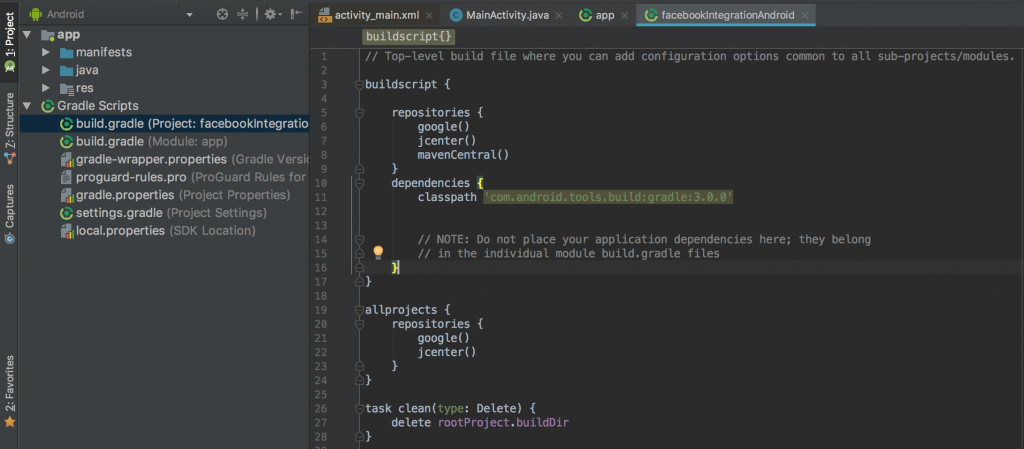
Similar way add implementation compile statement in build.gradle (Module: app).
implementation 'com.facebook.android:facebook-android-sdk:[5,6)' implementation 'com.squareup.picasso:picasso:2.5.2'
The package name is the application id from the manifest file which we need to put inside Facebook. Also need to add MainActivity full path for deep linking.
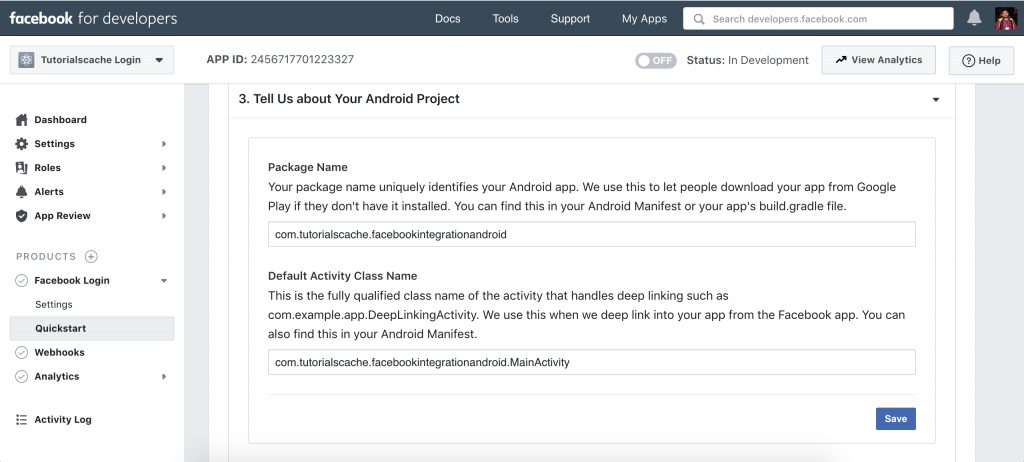
2. Key Hash
Mac OS
keytool -exportcert -alias androiddebugkey -keystore ~/.android/debug.keystore | openssl sha1 -binary | openssl base64
Windows
keytool -exportcert -alias androiddebugkey -keystore "C:\Users\USERNAME\.android\debug.keystore" | "PATH_TO_OPENSSL_LIBRARY\bin\openssl" sha1 -binary | "PATH_TO_OPENSSL_LIBRARY\bin\openssl" base64
Generating a Release Key Hash
keytool -exportcert -alias YOUR_RELEASE_KEY_ALIAS -keystore YOUR_RELEASE_KEY_PATH | openssl sha1 -binary | openssl base64
3. strings.xml
As moving forward we need to have facebook_app_id and fb_login_protocol_scheme under the quick start of Facebook Login.
<string name="facebook_app_id">APP_ID</string> <string name="fb_login_protocol_scheme">fb_login_protocol_id</string>
Colors.xml
<?xml version="1.0" encoding="utf-8"?> <resources> <color name="colorPrimary">#d11617</color> <color name="colorPrimaryDark">#B31718</color> <color name="colorAccent">#FF4081</color> </resources>
4. AndroidManifest.xml
Internet permissions are necessary to avoid network exceptions.
<uses-permission android:name="android.permission.INTERNET"/>
Facebook meta-data application under application as well.
<meta-data android:name="com.facebook.sdk.ApplicationId" android:value="@string/facebook_app_id"/> <activity android:name="com.facebook.FacebookActivity" android:configChanges= "keyboard|keyboardHidden|screenLayout|screenSize|orientation" android:label="@string/app_name" /> <activity android:name="com.facebook.CustomTabActivity" android:exported="true"> <intent-filter> <action android:name="android.intent.action.VIEW" /> <category android:name="android.intent.category.DEFAULT" /> <category android:name="android.intent.category.BROWSABLE" /> <data android:scheme="@string/fb_login_protocol_scheme" /> </intent-filter> </activity>
5. activity_main.xml
In activity_main.xml we are adding the login button ImageView and a few TextViews to show facebook returned users data like name, email, etc.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.tutorialscache.facebookintegrationandroid.MainActivity"> <ImageView android:id="@+id/fbIv" android:layout_width="140dp" android:layout_height="55dp" android:elevation="3dp" android:layout_centerHorizontal="true" android:layout_marginTop="80dp" android:layout_marginBottom="15dp" android:padding="3dp" android:scaleType="fitXY" android:src="@drawable/fb" /> <ImageView android:id="@+id/userIv" android:padding="10dp" android:src="@mipmap/ic_launcher" android:layout_centerHorizontal="true" android:layout_below="@id/fbIv" android:layout_width="100dp" android:layout_height="100dp" /> <TextView android:text="Email:" android:layout_below="@id/userIv" android:id="@+id/emailLabelTv" android:layout_centerHorizontal="true" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <TextView android:id="@+id/emailTv" android:textColor="@color/colorPrimary" android:layout_below="@+id/emailLabelTv" android:layout_centerHorizontal="true" android:layout_marginBottom="20dp" android:textSize="20dp" android:text="" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <TextView android:text="Name:" android:layout_below="@id/emailTv" android:id="@+id/nameLabelTv" android:layout_centerHorizontal="true" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <TextView android:id="@+id/nameTv" android:textColor="@color/colorPrimary" android:layout_below="@+id/nameLabelTv" android:layout_centerHorizontal="true" android:textSize="20dp" android:text="" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <ProgressBar android:id="@+id/mPb" android:visibility="gone" android:layout_below="@id/nameTv" android:layout_centerHorizontal="true" android:layout_marginTop="25dp" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </RelativeLayout>
6. MainActivity.java
MainActivity is the most important part of this tutorial. In this Activity, we will implement all code regarding interaction with the custom Facebook login button and as a result of this handle callback with the result.
FacebookSdk Initialisation, LoginManager, CallbackManager, GraphRequest, and onActivityResult are key components of this login implementation.
FacebookSdk.sdkInitialize is used to start facebook SDK so requests can be triggered.
package com.tutorialscache.facebookintegrationandroid; import android.app.Activity; import android.content.Context; import android.content.Intent; import android.graphics.Bitmap; import android.graphics.BitmapFactory; import android.net.Uri; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.ImageView; import android.widget.ProgressBar; import android.widget.TextView; import android.widget.Toast; import com.facebook.AccessToken; import com.facebook.CallbackManager; import com.facebook.FacebookCallback; import com.facebook.FacebookException; import com.facebook.FacebookSdk; import com.facebook.GraphRequest; import com.facebook.GraphResponse; import com.facebook.LoggingBehavior; import com.facebook.appevents.AppEventsLogger; import com.facebook.login.LoginManager; import com.facebook.login.LoginResult; import com.squareup.picasso.Picasso; import org.json.JSONException; import org.json.JSONObject; import java.util.Arrays; public class MainActivity extends AppCompatActivity { ProgressBar mPb; ImageView fbIv,userIv; TextView emailTv,nameTv; Context context; CallbackManager callbackManager; AccessToken access_token; GraphRequest request; private String email,facebook_uid,first_name,last_name,social_id,name,picture; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); context = this; setContentView(R.layout.activity_main); getViews(); } private void getViews() { mPb = findViewById(R.id.mPb); fbIv = findViewById(R.id.fbIv); nameTv = findViewById(R.id.nameTv); emailTv = findViewById(R.id.emailTv); userIv = findViewById(R.id.userIv); fbIv.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { facebookLoginSignup(); mPb.setVisibility(View.VISIBLE); } }); } private void facebookLoginSignup() { FacebookSdk.sdkInitialize(this.getApplicationContext()); if (BuildConfig.DEBUG) { FacebookSdk.setIsDebugEnabled(true); FacebookSdk.addLoggingBehavior(LoggingBehavior.INCLUDE_ACCESS_TOKENS); } LoginManager.getInstance().logInWithReadPermissions((Activity) context, Arrays.asList("email", "public_profile")); callbackManager = CallbackManager.Factory.create(); LoginManager.getInstance().registerCallback(callbackManager, new FacebookCallback<LoginResult>() { @Override public void onSuccess(LoginResult loginResult) { Log.d("response Success", "Login"); access_token = loginResult.getAccessToken(); Log.d("response access_token", access_token.toString()); request = GraphRequest.newMeRequest(AccessToken.getCurrentAccessToken(), new GraphRequest.GraphJSONObjectCallback() { @Override public void onCompleted(JSONObject object, GraphResponse response) { JSONObject json = response.getJSONObject(); try { if (json != null) { Log.d("response", json.toString()); try { email = json.getString("email"); emailTv.setText(email+""); } catch (Exception e) { Toast.makeText(context, "Sorry!!! Your email is not verified on facebook.", Toast.LENGTH_LONG).show(); return; } facebook_uid = json.getString("id"); social_id = json.getString("id"); first_name = json.getString("first_name"); last_name = json.getString("last_name"); name = json.getString("name"); nameTv.setText(name+""); picture = "https://graph.facebook.com/" + facebook_uid + "/picture?type=large"; Log.d("response", " picture"+picture); Picasso.with(context).load(picture).placeholder(R.mipmap.ic_launcher).into(userIv); mPb.setVisibility(View.GONE); } } catch (JSONException e) { e.printStackTrace(); Log.d("response problem", "problem" + e.getMessage()); } } }); Bundle parameters = new Bundle(); parameters.putString("fields", "id,name,first_name,last_name,link,email,picture"); request.setParameters(parameters); request.executeAsync(); } @Override public void onCancel() { Toast.makeText(context, "Login Cancel", Toast.LENGTH_LONG).show(); } @Override public void onError(FacebookException exception) { Toast.makeText(context, exception.getMessage(), Toast.LENGTH_LONG).show(); } }); } //region onResult @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { super.onActivityResult(requestCode, resultCode, data); callbackManager.onActivityResult(requestCode, resultCode, data); } }
Comments are closed.