Are you looking for a monetization solution for your android apps? Do you want to earn good revenue from your app? Then Google AdMob is the best solution for this purpose. It’s very easy to integrate AdMob ads into an android application. In this tutorial, we will learn to create Ad ids in the AdMob platform and then we will use these ids inside our android application.
We will use test ad ids inside our app which are provided by Google.
1. Create New Android Studio Project
Create a New Android Studio project and add the following code to your strings.xml file. It has test Ad Unit IDs provided by google which we will use in our app for testing. Once you are done with testing and ready to make the app live then you can replace these test IDs with your real AdMob IDs.
Strings.xml
<resources> <string name="app_name">AdMob Integration</string> <!-- sample Ad IDs and App ID--> <string name="admob_app_id">ca-app-pub-3940256099942544~3347511713</string> <string name="banner_ad">ca-app-pub-3940256099942544/6300978111</string> <string name="Interstitial">ca-app-pub-3940256099942544/1033173712</string> <string name="rewarded_video">ca-app-pub-3940256099942544/8691691433</string> <string name="native_advanced">ca-app-pub-3940256099942544/2247696110</string> </resources>
2. Mobile Ads SDK
Open project-level build.gradle the project-level file and look for allprojects section. Add google() under repositories if not present there it will help to add Gradle dependency which points to Google’s Maven.
allprojects { repositories { google() jcenter() } }
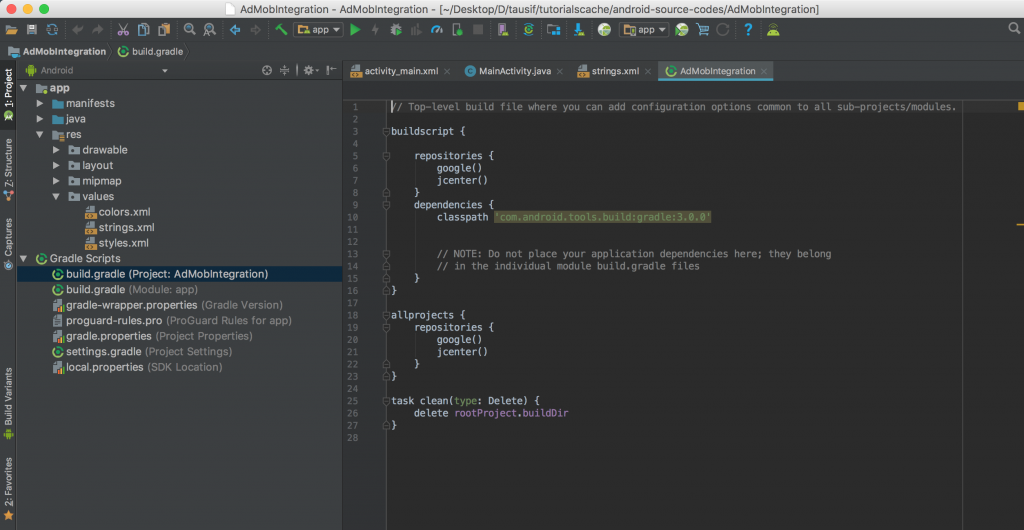
Next, open the build.gradle(Modle app) and add the following code under the dependencies section.
implementation 'com.google.android.gms:play-services-ads:11.8.0'
3. AndroidManifest.xml Permissions & Meta-data
Internet permissions to show because ads are visible using the internet. Add the following line of code to your manifest file. As a result of this, we will not have an internet permissions warning.
<uses-permission android:name="android.permission.INTERNET"/>
Next, we need to add our Admob App ID under application within a <meta-data>. You will replace the value of this meta-data with your on App ID. Here is what AndroidManifest will look like after adding application ID meta-data.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.tutorialscache.admobintegration"> <uses-permission android:name="android.permission.INTERNET"/> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> <meta-data android:name="com.google.android.gms.ads.APPLICATION_ID" android:value="@string/admob_app_id"> </meta-data> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
4. Initialise Ads SDK
Before loading ads, we need to initialize ads SDK. To accomplish this task we will create a new GlobalClass and extend that with the application. This initialization needs to be done once in the app that’s why we are adding this in an application. GlobalClass will look like this.
package com.tutorialscache.admobintegration; import android.app.Application; import com.google.android.gms.ads.MobileAds; public class GlobalClass extends Application { @Override public void onCreate() { super.onCreate(); MobileAds.initialize(this); } }
Finally, for Ads initialization, we need to add GlobaClass in the AndroidManifest file as a name.
<!-- AndroidManifest.xm --> <application android:name=".GlobalClass" ..> </application>
5. colors.xml
Add the following code to your colors.xml file.
<?xml version="1.0" encoding="utf-8"?> <resources> <color name="colorPrimary">#d11617</color> <color name="colorPrimaryDark">#B31718</color> <color name="colorAccent">#FF4081</color> </resources>
6. activity_main.xml
In activity_main we will add two buttons to show interstitial Ads and video rewarded ads. It has two most important attributes of adSize and adUnitId. AdUnitId is linked from strings.xml banner ad id.
View type AdView is used to display BANNER Ads.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" xmlns:ads="http://schemas.android.com/apk/res-auto" tools:context="com.tutorialscache.admobintegration.MainActivity"> <TextView android:id="@+id/title" android:text="Google Ads" android:textColor="@color/colorPrimary" android:layout_centerHorizontal="true" android:textSize="30dp" android:layout_marginTop="20dp" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <Button android:id="@+id/interstitialAdBtn" android:layout_below="@id/title" android:layout_marginTop="120dp" android:layout_centerHorizontal="true" android:text="Interstitial Ad" android:background="@color/colorPrimary" android:textColor="#FFFFFF" android:layout_width="200dp" android:layout_height="wrap_content" /> <Button android:layout_below="@id/interstitialAdBtn" android:layout_marginTop="50dp" android:layout_centerHorizontal="true" android:text="Video Reward" android:background="@color/colorPrimary" android:textColor="#FFFFFF" android:layout_width="200dp" android:layout_height="wrap_content" /> <com.google.android.gms.ads.AdView android:id="@+id/bannerAdView" ads:adUnitId="@string/banner_ad" ads:adSize="BANNER" android:layout_alignParentBottom="true" android:layout_centerHorizontal="true" android:layout_width="wrap_content" android:layout_height="wrap_content"> </com.google.android.gms.ads.AdView> </RelativeLayout>
Related: How to use Material Design icons in Android.
8. MainActivity.java – BANNER AD
For displaying the banner id at the main activity we need to link bannerAdView and then create an AdMob request object to loadAd on bannerAdView.
Banner AdView also has callbacks that provide the status of the ad whether it’s successfully loaded, closed, clicked, or failed to close.
package com.tutorialscache.admobintegration; import android.content.Context; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.Toast; import com.google.android.gms.ads.AdListener; import com.google.android.gms.ads.AdRequest; import com.google.android.gms.ads.AdView; import com.google.android.gms.ads.InterstitialAd; public class MainActivity extends AppCompatActivity implements View.OnClickListener{ AdView bannerAdView; AdRequest adRequestObj; Context context; Button interstitialAdBtn,videoRewardAdBtn; InterstitialAd interstitialAd; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); context=this; setContentView(R.layout.activity_main); //link views from activity_main.xml getViews(); //ad request object adRequestObj = new AdRequest.Builder() .addTestDevice(AdRequest.DEVICE_ID_EMULATOR) // Device Id for testing from logcat : screenshot is added below .addTestDevice("F9B3B4B772E94A45CE7AECF1E0EE24C7") .build(); bannerAdView.setAdListener(new AdListener(){ @Override public void onAdLoaded() { super.onAdLoaded(); } @Override public void onAdClosed() { super.onAdClosed(); Toast.makeText(context,"Ad closed",Toast.LENGTH_SHORT).show(); } @Override public void onAdClicked() { super.onAdClicked(); } @Override public void onAdFailedToLoad(int i) { super.onAdFailedToLoad(i); Toast.makeText(context,"Failed to load ad: Error"+i,Toast.LENGTH_SHORT).show(); } @Override public void onAdImpression() { super.onAdImpression(); } @Override public void onAdLeftApplication() { super.onAdLeftApplication(); } @Override public void onAdOpened() { super.onAdOpened(); } }); bannerAdView.loadAd(adRequestObj); } private void getViews() { bannerAdView = findViewById(R.id.bannerAdView); interstitialAdBtn = findViewById(R.id.interstitialAdBtn); interstitialAdBtn.setOnClickListener(this); videoRewardAdBtn = findViewById(R.id.videoRewardAdBtn); videoRewardAdBtn.setOnClickListener(this); } @Override public void onClick(View view) { int id = view.getId(); switch (id){ case R.id.interstitialAdBtn: showInterstitialAd(); break; case R.id.videoRewardAdBtn: //un comment below line after step 10 //startActivity(new Intent(context, VideoRewardAdActivity.class)); break; } } private void showInterstitialAd() { } @Override public void onPause() { if (bannerAdView != null) { bannerAdView.pause(); } super.onPause(); } @Override public void onResume() { super.onResume(); if (bannerAdView != null) { bannerAdView.resume(); } } @Override public void onDestroy() { if (bannerAdView != null) { bannerAdView.destroy(); } super.onDestroy(); } }

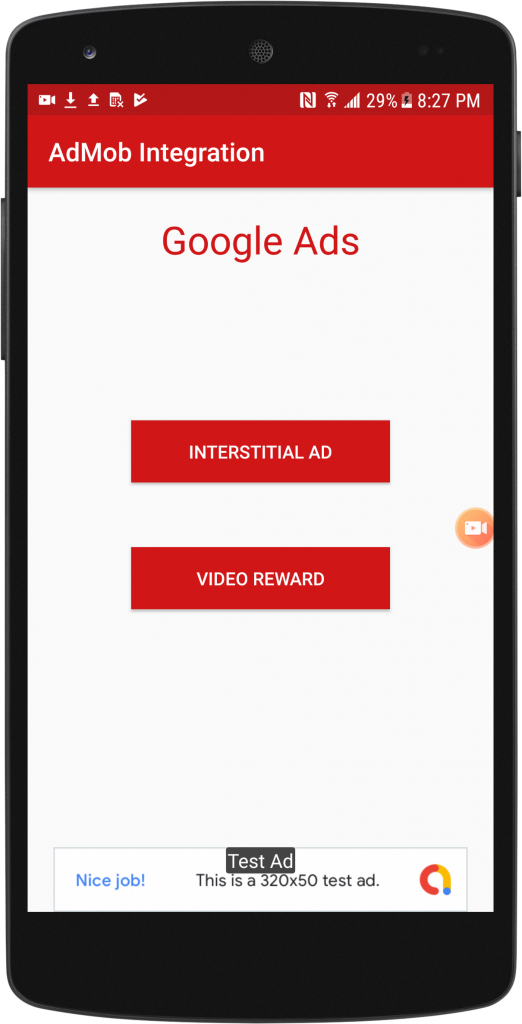
9. Interstitial Ad
Interstitial Ads are also called full-screen ads. These ads are created dynamically so we don’t need to add any code under the activity_main.xml file. We will implement an interstitial ad function inside our previously created function showInterstitialAd(). Here is the implementation.
private void showInterstitialAd() { interstitialAd = new InterstitialAd(context); interstitialAd.setAdUnitId(getString(R.string.Interstitial)); AdRequest interstitialAdRequest = new AdRequest.Builder() .build(); // Load ads into Interstitial Ads interstitialAd.loadAd(interstitialAdRequest); interstitialAd.setAdListener(new AdListener() { public void onAdLoaded() { if (interstitialAd.isLoaded()) { interstitialAd.show(); } } }); }
One of the most important things about interstitial ads is accidental clicks. To avoid this we always need to implement actions under its callbacks. Suppose if you want to open a new activity then its intent code should be implemented under its closed
interstitialAd.setAdListener(new AdListener() { @Override public void onAdLoaded() { super.onAdLoaded(); //Ads loaded } @Override public void onAdClosed() { super.onAdClosed(); //Ads closed //open new activity / perform action } @Override public void onAdFailedToLoad(int errorCode) { super.onAdFailedToLoad(errorCode); //Ads couldn't loaded } });
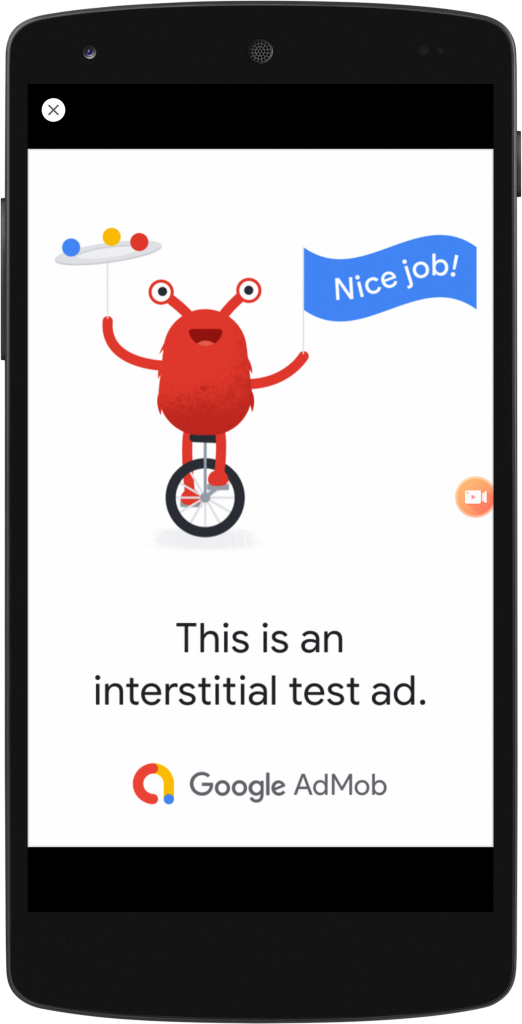
Complete MainActivity.java code
package com.tutorialscache.admobintegration; import android.content.Context; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.Toast; import com.google.android.gms.ads.AdListener; import com.google.android.gms.ads.AdRequest; import com.google.android.gms.ads.AdView; import com.google.android.gms.ads.InterstitialAd; public class MainActivity extends AppCompatActivity implements View.OnClickListener{ AdView bannerAdView; AdRequest adRequestObj; Context context; Button interstitialAdBtn,videoRewardAdBtn; InterstitialAd interstitialAd; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); context=this; setContentView(R.layout.activity_main); //link views from activity_main.xml getViews(); //ad request object adRequestObj = new AdRequest.Builder() .addTestDevice(AdRequest.DEVICE_ID_EMULATOR) // Device Id for testing from logcat : screenshot is added below .addTestDevice("F9B3B4B772E94A45CE7AECF1E0EE24C7") .build(); bannerAdView.setAdListener(new AdListener(){ @Override public void onAdLoaded() { super.onAdLoaded(); } @Override public void onAdClosed() { super.onAdClosed(); Toast.makeText(context,"Ad closed",Toast.LENGTH_SHORT).show(); } @Override public void onAdClicked() { super.onAdClicked(); } @Override public void onAdFailedToLoad(int i) { super.onAdFailedToLoad(i); Toast.makeText(context,"Failed to load ad: Error"+i,Toast.LENGTH_SHORT).show(); } @Override public void onAdImpression() { super.onAdImpression(); } @Override public void onAdLeftApplication() { super.onAdLeftApplication(); } @Override public void onAdOpened() { super.onAdOpened(); } }); bannerAdView.loadAd(adRequestObj); } private void getViews() { bannerAdView = findViewById(R.id.bannerAdView); interstitialAdBtn = findViewById(R.id.interstitialAdBtn); interstitialAdBtn.setOnClickListener(this); videoRewardAdBtn = findViewById(R.id.videoRewardAdBtn); videoRewardAdBtn.setOnClickListener(this); } @Override public void onClick(View view) { int id = view.getId(); switch (id){ case R.id.interstitialAdBtn: showInterstitialAd(); break; case R.id.videoRewardAdBtn: //startActivity(new Intent(context,VideoRewardAdActivity.class)); break; } } private void showInterstitialAd() { interstitialAd = new InterstitialAd(context); interstitialAd.setAdUnitId(getString(R.string.Interstitial)); AdRequest interstitialAdRequest = new AdRequest.Builder() .build(); interstitialAd.setAdListener(new AdListener() { @Override public void onAdLoaded() { super.onAdLoaded(); //Ads loaded } @Override public void onAdClosed() { super.onAdClosed(); //Ads closed //open new activity / perform action } @Override public void onAdFailedToLoad(int errorCode) { super.onAdFailedToLoad(errorCode); //Ads couldn't loaded } }); // Load ads into Interstitial Ads interstitialAd.loadAd(interstitialAdRequest); interstitialAd.setAdListener(new AdListener() { public void onAdLoaded() { if (interstitialAd.isLoaded()) { interstitialAd.show(); } } }); } @Override public void onPause() { if (bannerAdView != null) { bannerAdView.pause(); } super.onPause(); } @Override public void onResume() { super.onResume(); if (bannerAdView != null) { bannerAdView.resume(); } } @Override public void onDestroy() { if (bannerAdView != null) { bannerAdView.destroy(); } super.onDestroy(); } }
10. Video Rewarded Ad
To display a Video rewarded Ad create a new activity VideoRewardAdActivity and add the following code.
package com.tutorialscache.admobintegration; import android.content.Context; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log; import android.widget.Toast; import com.google.android.gms.ads.AdRequest; import com.google.android.gms.ads.MobileAds; import com.google.android.gms.ads.reward.RewardItem; import com.google.android.gms.ads.reward.RewardedVideoAd; import com.google.android.gms.ads.reward.RewardedVideoAdListener; public class VideoRewardAdActivity extends AppCompatActivity { RewardedVideoAd rewardedVideoAd; Context context; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); context=this; setContentView(R.layout.activity_video_reward_ad); rewardedVideoAd = MobileAds.getRewardedVideoAdInstance(context); rewardedVideoAd.loadAd(getString(R.string.rewarded_video), new AdRequest.Builder().addTestDevice(AdRequest.DEVICE_ID_EMULATOR) // Device Id for testing from logcat .addTestDevice("F9B3B4B772E94A45CE7AECF1E0EE24C7").build()); rewardedVideoAd.setRewardedVideoAdListener(new RewardedVideoAdListener() { @Override public void onRewarded(RewardItem rewardItem) { Log.d("response", "Type : " + rewardItem.getType() + " Price: " + rewardItem.getAmount()); } @Override public void onRewardedVideoAdLeftApplication() { Log.d("response", "rewarded ad left app"); } @Override public void onRewardedVideoAdClosed() { Log.d("response","video ad closed"); finish(); } @Override public void onRewardedVideoAdFailedToLoad(int errorCode) { Log.d("response","video ad failed to load"+errorCode); } @Override public void onRewardedVideoAdLoaded() { Log.d("response","video ad loaded"); if (rewardedVideoAd.isLoaded()) { rewardedVideoAd.show(); } } @Override public void onRewardedVideoAdOpened() { Log.d("response","video ad opened"); } @Override public void onRewardedVideoStarted() { Log.d("response","rewarded video ad started"); } }); } @Override public void onResume() { rewardedVideoAd.resume(context); super.onResume(); } @Override public void onPause() { rewardedVideoAd.pause(context); super.onPause(); } @Override public void onDestroy() { rewardedVideoAd.destroy(context); super.onDestroy(); } }
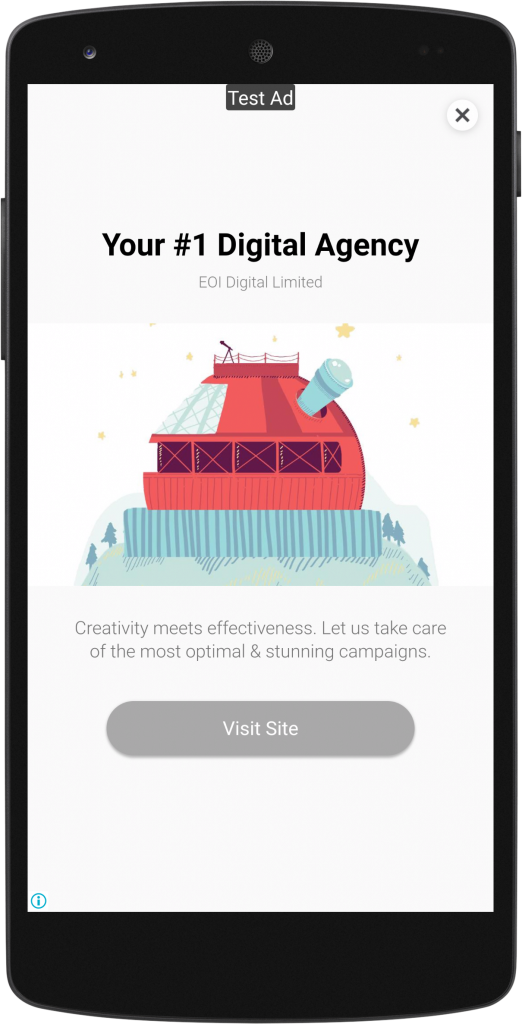
Comments are closed.