Checkbox Unchecked: It’s a common problem with beginners in android application development once they implement a checkbox in ListView that automatically gets unchecked while scrolling. In this tutorial, we will learn how to avoid this problem. We will implement a custom adapter with a custom row having checkboxes and titles.
1. App name, Package name, and Project location.
Create a new project with the name CheckBoxListView and package name com.tutorialscache.checkboxchecklist.
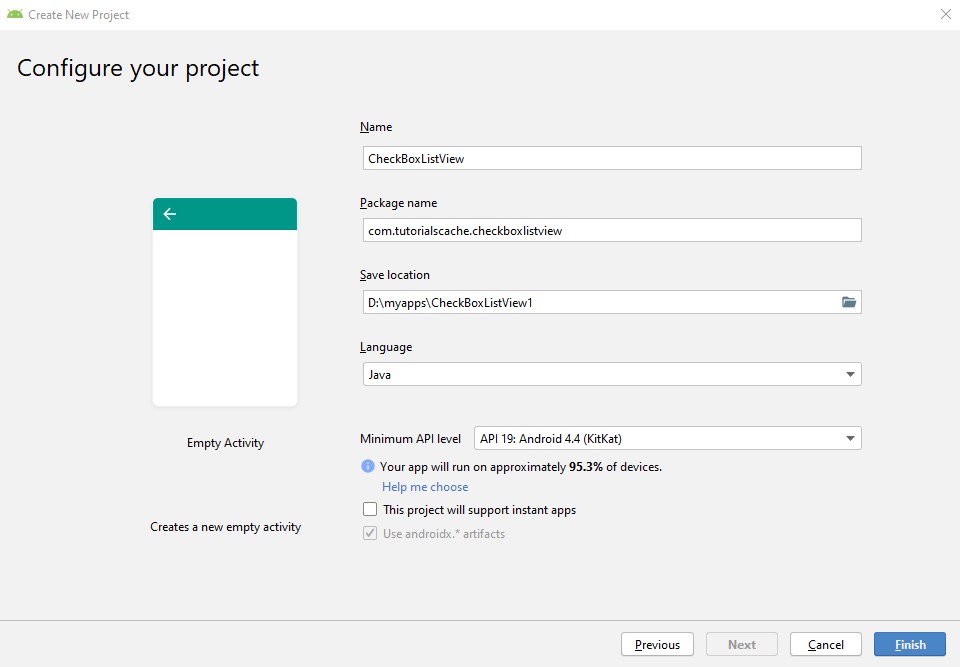
2. Choose Empty Activity
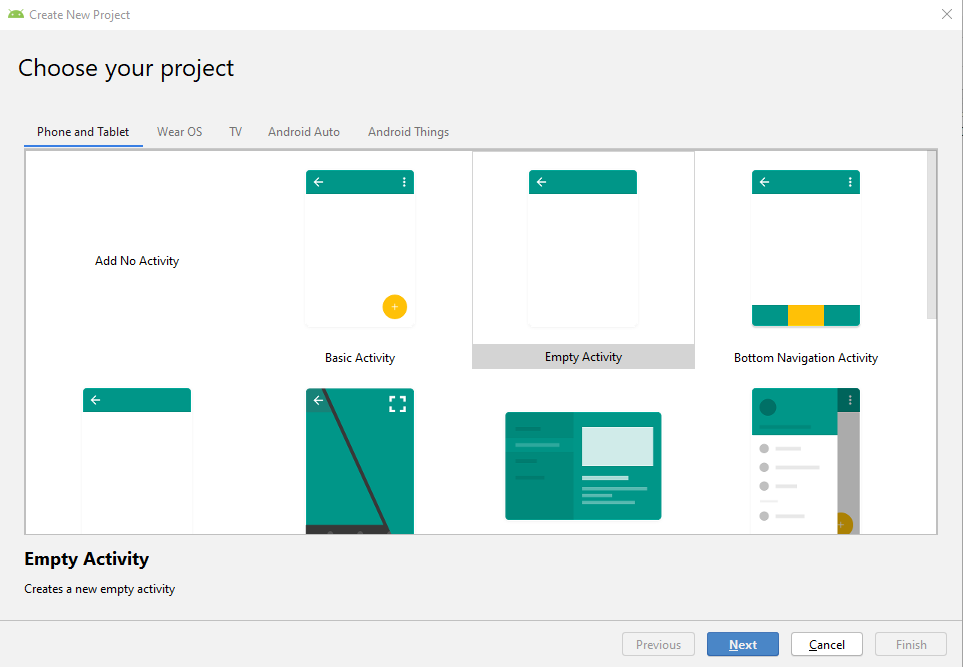
3. activity_main.xml
Add a Custom ListView using BaseAdapter inside activity_main.xml and assign id listView.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#e9e9e9" tools:context="com.tutorialscache.checkboxlistview.MainActivity"> <ListView android:id="@+id/listview_list" android:layout_width="match_parent" android:layout_height="match_parent"> </ListView> </LinearLayout>
4. Custom Row movie_row.xml
Right-click on layout and create new > Layout Resource File. This row will be used by the adapter to render each movie item.
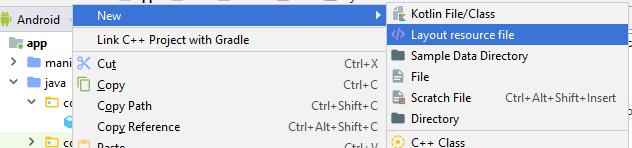
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="10dp" android:background="#f7f7f7"> <TextView android:id="@+id/movieNameTv" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginStart="10dp" android:textSize="20sp" android:textStyle="bold" android:textColor="#000"/> <CheckBox android:id="@+id/checkbox" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentEnd="true" /> </RelativeLayout>
5. Create model MovieModel.java
5.1 Right-click on the Package name > New > Java Class and give the model name MovieModel and click Ok.
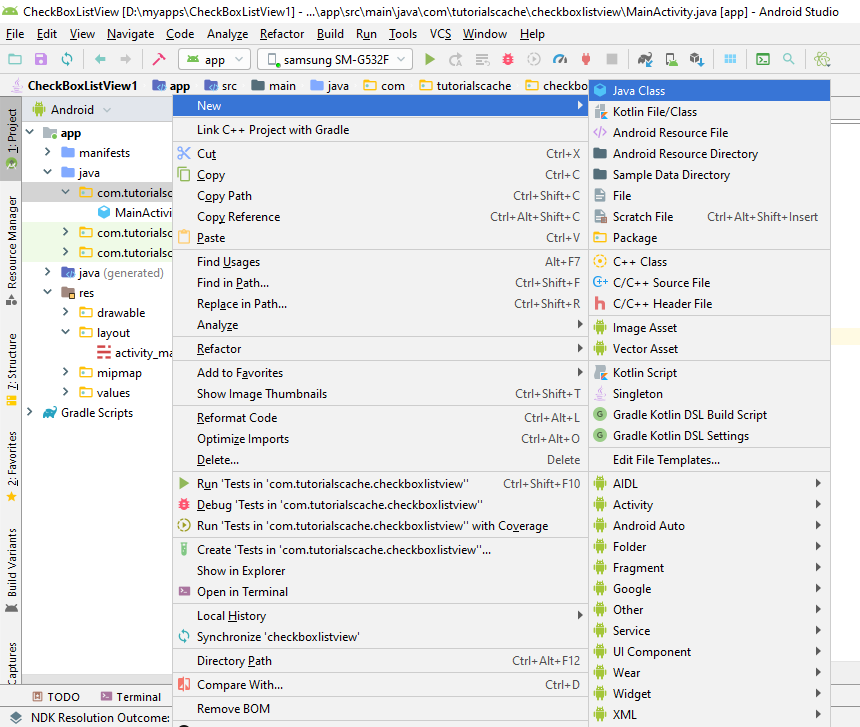
5.2 Create Getters and Setters
Press Alt +Insert to Generate Getter and Setter.
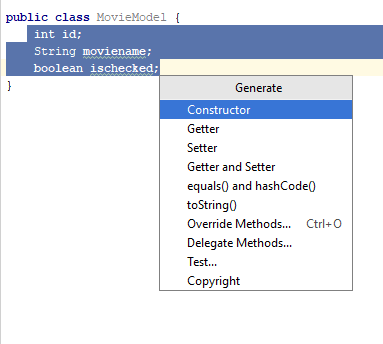
package com.tutorialscache.checkboxlistview; public class MoviesModel { int id; String moviename; boolean ischecked; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getMoviename() { return moviename; } public void setMoviename(String moviename) { this.moviename = moviename; } public boolean isIschecked() { return ischecked; } public void setIschecked(boolean ischecked) { this.ischecked = ischecked; } }
6. MoviesAdapter.java
Create a new java class and name as MoviesAdapter by clicking on package name > New > Java Class. Extend with BaseAdapter following cmd+insert to implement methods.
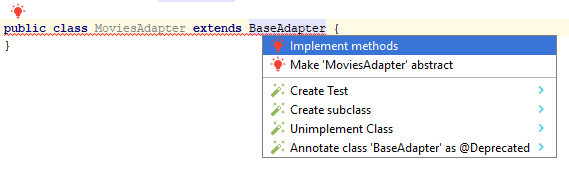
Full MoviesAdapter.java Code.
Tag is one of the most important things in android development to get the position of clicked view. In our case, we will set a position as a tag on our checkbox and we will get tag when the checkbox is clicked. As a result, we will be able to get the exact position of the clicked view which will help to change isChecked state to true/false.
package com.tutorialscache.checkboxlistview; import android.content.Context; import android.util.Log; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.BaseAdapter; import android.widget.CheckBox; import android.widget.TextView; import java.util.ArrayList; public class MoviesAdapter extends BaseAdapter { private Context context; private ArrayList<MovieModel> moviesData; private LayoutInflater layoutInflater; private MovieModel movieModel; public MoviesAdapter(Context context, ArrayList<MovieModel> moviesData) { this.context = context; this.moviesData = moviesData; layoutInflater= (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE); } @Override public int getCount() {; return moviesData.size(); } @Override public Object getItem(int position) { return moviesData.get(position); } @Override public long getItemId(int position) { return moviesData.get(position).getId(); } @Override public View getView(int position, View view, ViewGroup parent) { View rowView=view; if(rowView==null) { rowView=layoutInflater.inflate(R.layout.movies_row,parent,false); } TextView moviesName=(TextView)rowView.findViewById(R.id.movieNameTv); CheckBox checkBox=(CheckBox)rowView.findViewById(R.id.checkbox); movieModel = moviesData.get(position); moviesName.setText(movieModel.getMoviename()); checkBox.setChecked(movieModel.isIschecked()); // Tag is important to get position clicked checkbox checkBox.setTag(position); checkBox.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { int currentPos = (int) v.getTag(); boolean isChecked = false; if (moviesData.get(currentPos).isIschecked()==false){ isChecked=true; } Log.d("response ",currentPos+ " "+isChecked); moviesData.get(currentPos).setIschecked(isChecked); notifyDataSetChanged(); } }); return rowView; } }
7. MainActivity.java
Full MainActivity.java Code.
package com.tutorialscache.checkboxlistview; import androidx.appcompat.app.AppCompatActivity; import android.content.Context; import android.os.Bundle; import android.widget.ListView; import java.util.ArrayList; public class MainActivity extends AppCompatActivity { ListView mlistView; Context context; ArrayList<MovieModel> moviesData; MoviesAdapter moviesAdapter; MovieModel movieModel; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); context = this; setContentView(R.layout.activity_main); //getviews mlistView = findViewById(R.id.listview_list); moviesData = new ArrayList<>(); //add Countries Data addMoviesData(); moviesAdapter =new MoviesAdapter(context,moviesData); mlistView.setAdapter(moviesAdapter); } private void addMoviesData() { //Movie1 movieModel =new MovieModel(); movieModel.setId(1); movieModel.setMoviename("Avangers"); movieModel.setIschecked(false); moviesData.add(movieModel); //Movie2 movieModel =new MovieModel(); movieModel.setId(2); movieModel.setMoviename("InfinityWar"); movieModel.setIschecked(false); moviesData.add(movieModel); //Movie3 movieModel =new MovieModel(); movieModel.setId(3); movieModel.setMoviename("Need For Speed"); movieModel.setIschecked(false); moviesData.add(movieModel); //Movie4 movieModel =new MovieModel(); movieModel.setId(4); movieModel.setMoviename("Deadpool"); movieModel.setIschecked(false); moviesData.add(movieModel); //Movie5 movieModel =new MovieModel(); movieModel.setId(5); movieModel.setMoviename("War"); movieModel.setIschecked(false); moviesData.add(movieModel); //Movie6 movieModel =new MovieModel(); movieModel.setId(6); movieModel.setMoviename("Twilight"); movieModel.setIschecked(false); moviesData.add(movieModel); //Movie7 movieModel =new MovieModel(); movieModel.setId(7); movieModel.setMoviename("Twilight saga"); movieModel.setIschecked(false); moviesData.add(movieModel); //Movie8 movieModel =new MovieModel(); movieModel.setId(8); movieModel.setMoviename("ABCD"); movieModel.setIschecked(false); moviesData.add(movieModel); //Movie9 movieModel =new MovieModel(); movieModel.setId(9); movieModel.setMoviename("ABCD2"); movieModel.setIschecked(false); moviesData.add(movieModel); //Movie10 movieModel =new MovieModel(); movieModel.setId(10); movieModel.setMoviename("Dhoom"); movieModel.setIschecked(false); moviesData.add(movieModel); //Movie11 movieModel =new MovieModel(); movieModel.setId(11); movieModel.setMoviename("See"); movieModel.setIschecked(false); moviesData.add(movieModel); //Movie12 movieModel =new MovieModel(); movieModel.setId(12); movieModel.setMoviename("Batman"); movieModel.setIschecked(false); moviesData.add(movieModel); //Movie13 movieModel =new MovieModel(); movieModel.setId(13); movieModel.setMoviename("Man Of Steel"); movieModel.setIschecked(false); moviesData.add(movieModel); //Movie14 movieModel =new MovieModel(); movieModel.setId(14); movieModel.setMoviename("Age of Altron"); movieModel.setIschecked(false); moviesData.add(movieModel); //Movie15 movieModel =new MovieModel(); movieModel.setId(15); movieModel.setMoviename("Ender Games"); movieModel.setIschecked(false); moviesData.add(movieModel); //Movie16 movieModel =new MovieModel(); movieModel.setId(16); movieModel.setMoviename("Speed"); movieModel.setIschecked(false); moviesData.add(movieModel); } }
Final Output of the app
Relevant: How to integrate Google AdMob into Android Application.
Comments are closed.