How to build REST API? How to connect an android application with PHP and MySQL database? What is JSON? How to make POST Server requests in android? How to build login signup in android using PHP and MySQL? These are the main questions that came to mind once we think about connecting the android application with the server. In this tutorial, we will try to answer these questions and will make a complete user registration system.
If you are new to android development then you must first learn how to parse using this tutorial. JSON Parsing in Android.
Let’s get started with creating a database and REST API script.
1. Create a Database
Start your server and create a new database with the name android_app.
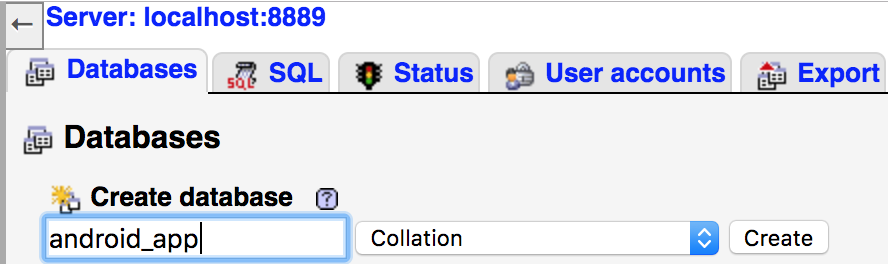
After creating the database now we need to create a user table. Copy the below code and run it in the SQL section of your server.
2. Create Users Table
-- -- Table structure for table `users` -- CREATE TABLE `users` ( `id` int(11) NOT NULL, `name` varchar(255) NOT NULL, `email` varchar(255) NOT NULL, `password` varchar(255) NOT NULL, `created_at` datetime NOT NULL DEFAULT CURRENT_TIMESTAMP ) ENGINE=InnoDB DEFAULT CHARSET=utf8; -- -- Indexes for dumped tables -- -- -- Indexes for table `users` -- ALTER TABLE `users` ADD PRIMARY KEY (`id`); -- -- AUTO_INCREMENT for dumped tables -- -- -- AUTO_INCREMENT for table `users` -- ALTER TABLE `users` MODIFY `id` int(11) NOT NULL AUTO_INCREMENT;
After creating the user’s table this is how it will look in the Structure view.
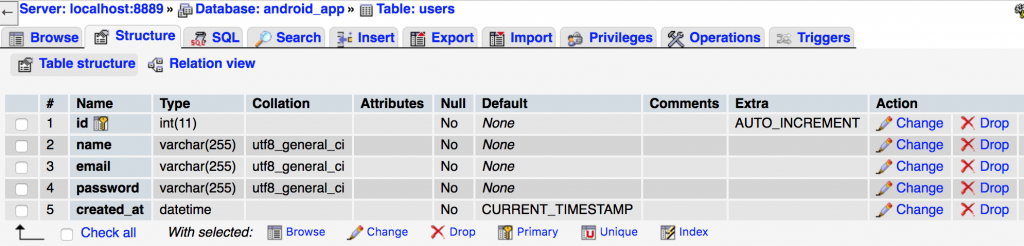
3. PHP REST API
Now we need to write a simple PHP API that will be used to exchange data between the app and web server. Follow below simple steps to create API.
- Create a new folder named androidapp inside htdocs/www.
- Create a new PHP file under androidAppFolder and name it api.php
To avoid any complexity of files we will use only one api.php file for the whole REST API functionality. You can copy the below code and paste it into your api.php file. It has comments at each step so as a result of that you can understand it easily.
Api.php
<?php // step 1: connect to database // mysqli_connect function has 4 params (host,user name, password,database_name) $db_con = mysqli_connect("localhost","root","root","android_app"); $response = array(); header('Content-Type: application/json'); if(mysqli_connect_errno()) { $response["error"] = TRUE; $response["message"] ="Faild to connect to database"; echo json_encode($response); exit; } if(isset($_POST["type"]) && ($_POST["type"]=="signup") && isset($_POST["name"]) && isset($_POST["email"]) && isset($_POST["password"])){ // signup user $name = $_POST["name"]; $email = $_POST["email"]; $password = md5($_POST["password"]); //check user email whether its already regsitered $checkEmailQuery = "select * from users where email = '$email'"; $result = mysqli_query($db_con,$checkEmailQuery); // print_r($result); exit; if($result->num_rows>0){ $response["error"] = TRUE; $response["message"] ="Sorry email already found."; echo json_encode($response); exit; }else{ $signupQuery = "INSERT INTO users(name,email,password) values('$name','$email','$password')"; $signupResult = mysqli_query($db_con,$signupQuery); if($signupResult){ // Get Last Inserted ID $id = mysqli_insert_id($db_con); // Get User By ID $userQuery = "SELECT id,name,email FROM users WHERE id = ".$id; $userResult = mysqli_query($db_con,$userQuery); $user = mysqli_fetch_assoc($userResult); $response["error"] = FALSE; $response["message"] = "Successfully signed up."; $response["user"] = $user; echo json_encode($response); exit; }else{ $response["error"] = TRUE; $response["message"] ="Unable to signup try again later."; echo json_encode($response); exit; } } }else if(isset($_POST["type"]) && ($_POST["type"]=="login") && isset($_POST["email"]) && isset($_POST["password"])){ //login user $email = $_POST["email"]; $password = md5($_POST["password"]); $userQuery = "select id,name,email from users where email = '$email' && password = '$password'"; $result = mysqli_query($db_con,$userQuery); // print_r($result); exit; if($result->num_rows==0){ $response["error"] = TRUE; $response["message"] ="user not found or Invalid login details."; echo json_encode($response); exit; }else{ $user = mysqli_fetch_assoc($result); $response["error"] = FALSE; $response["message"] = "Successfully logged in."; $response["user"] = $user; echo json_encode($response); exit; } }else { // Invalid parameters $response["error"] = TRUE; $response["message"] ="Invalid parameters"; echo json_encode($response); exit; } ?>
You can try running this code using postman.
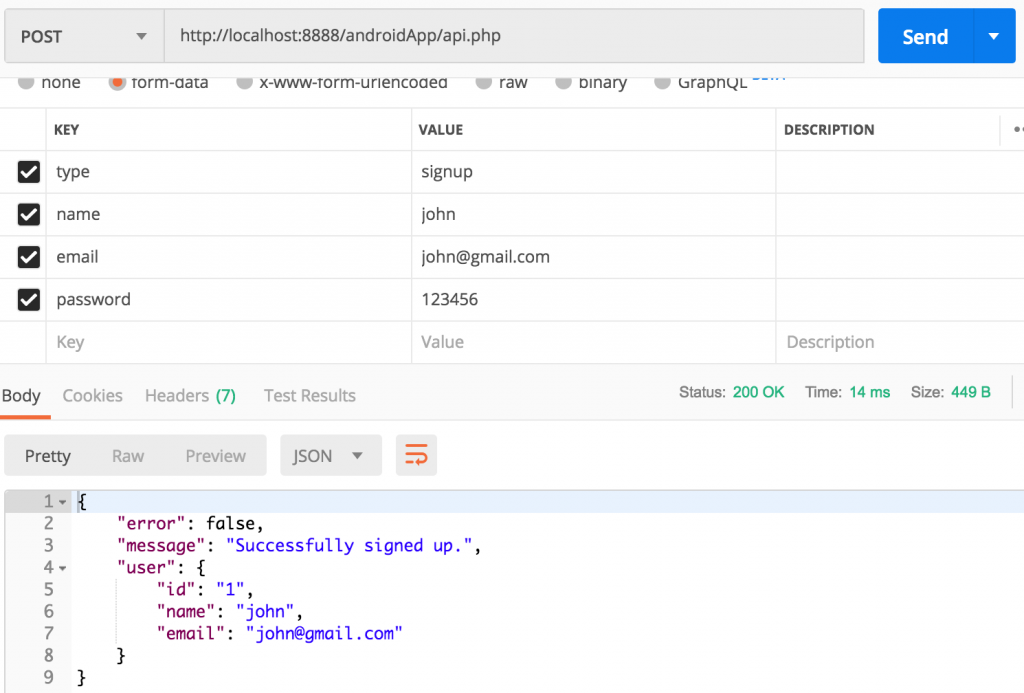
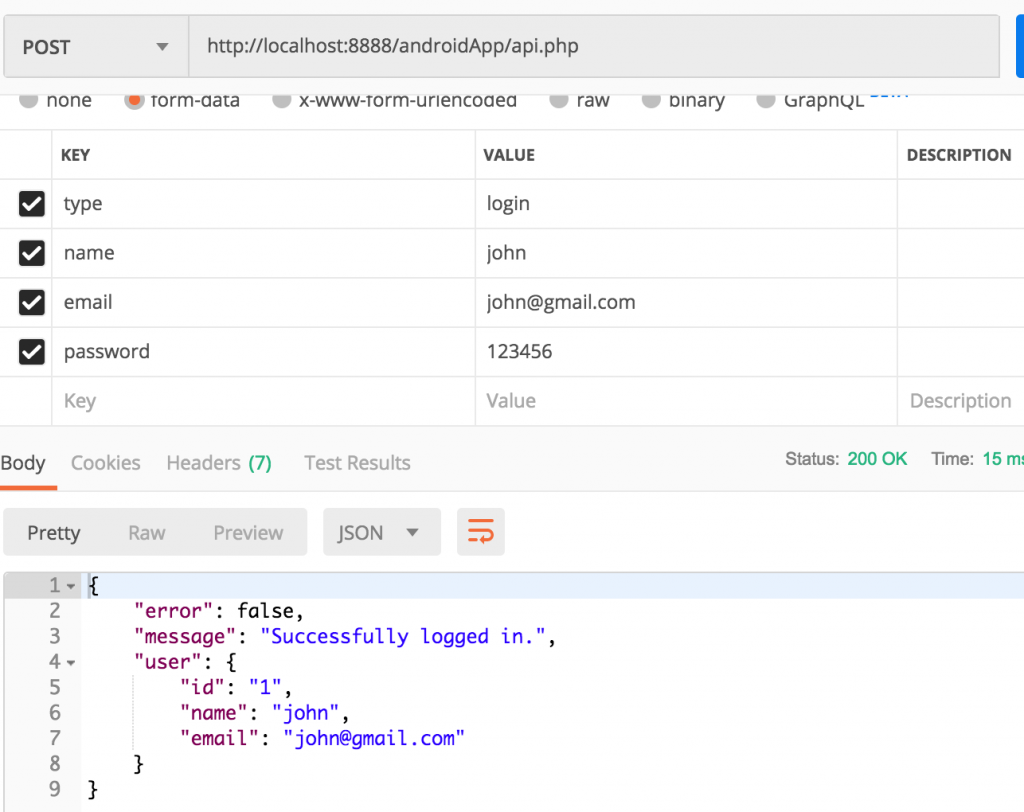
The most important parameter is the type which actually helps to differentiate between login and signup APIs.
This is all we need to do on the server side now we need to start working on Android App using Android Studio.
4. Create a New Android Studio Project
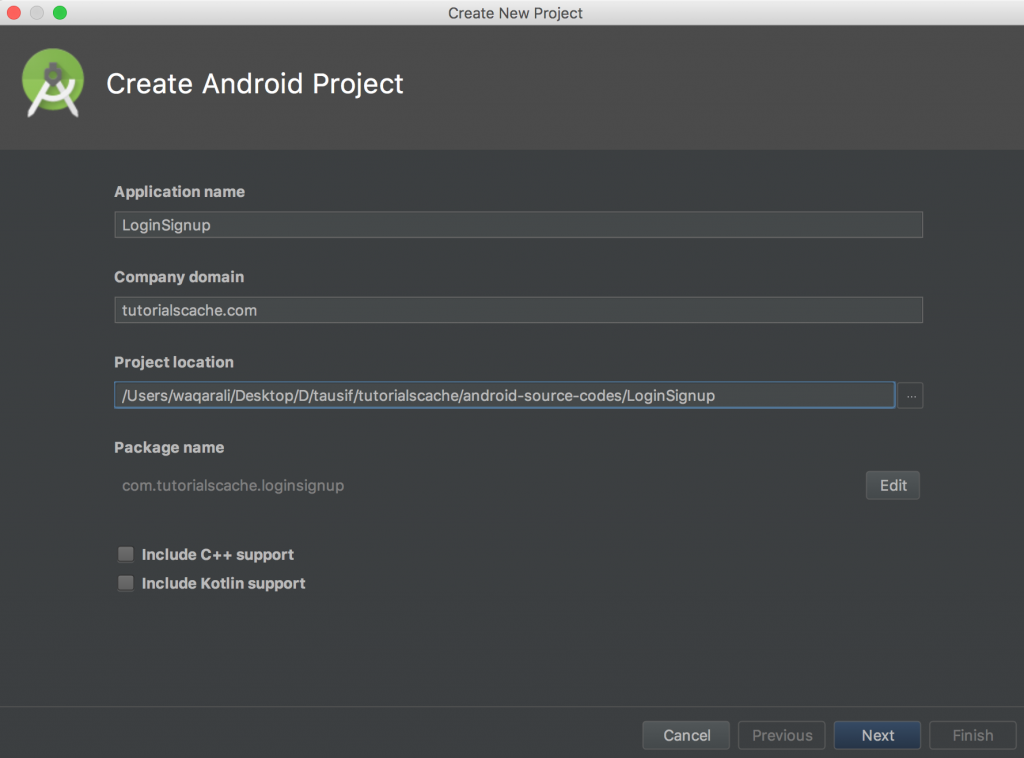
Add the following code to colors.xml
<?xml version="1.0" encoding="utf-8"?> <resources> <color name="colorPrimary">#d11617</color> <color name="colorPrimaryDark">#B31718</color> <color name="colorAccent">#FF4081</color> <color name="white">#FFFFFF</color> </resources>
After creating and new Android Studio Project now we need to create 4 more activities.
- SplashActivity
- LoginActivity
- SignupActivity
- HomeActivity
5. AndroidManifest.xml INTERNET Permissions
<uses-permission android:name="android.permission.INTERNET"/>
6. Third-party libraries Loopj & Picasso.
We will use loopj to access data from URL and Picasso to load images from URL in build.gradle (Module: app)
implementation 'com.loopj.android:android-async-http:1.4.9' implementation 'com.squareup.picasso:picasso:2.5.2'
Module: App complete code
apply plugin: 'com.android.application' android { compileSdkVersion 27 defaultConfig { applicationId "com.tutorialscache.loginsignup" minSdkVersion 14 targetSdkVersion 27 versionCode 1 versionName "1.0" testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner" } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro' } } } dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) implementation 'com.android.support:appcompat-v7:27.1.1' implementation 'com.android.support:support-annotations:27.1.1' implementation 'com.android.support.constraint:constraint-layout:1.1.3' testImplementation 'junit:junit:4.12' androidTestImplementation 'com.android.support.test:runner:1.0.2' androidTestImplementation 'com.android.support.test.espresso:espresso-core:3.0.2' implementation 'com.loopj.android:android-async-http:1.4.9' implementation 'com.squareup.picasso:picasso:2.5.2' }
7. GlobalClass.java
Create a new class GlobalClass and extend it with Application. This class instance will help us to access the BASE URL and also gives us an application instance.
import android.app.Application; public class GlobalClass extends Application { public static final String BASE_URL = "http://192.168.18.8:8888/androidApp/api.php"; // public static final String BASE_URL = "http:/localhost:8888/androidApp/api.php"; private static GlobalClass singleton; @Override public void onCreate() { super.onCreate(); singleton = this; } public static GlobalClass getInstance() { return singleton; } }
To launch GlobalClass at the start of the application add the application name in the name attribute in <application> of AndroidManifest.xml
<application android:name=".GlobalClass"
8. WebReq.java
Create a new Class name WebReq and follow the code. WebReq class is used to send requests on the server. It has a GET/POST Method to return JSON data from a given URL.
package com.tutorialscache.loginsignup; import android.content.Context; import android.util.Log; import com.loopj.android.http.AsyncHttpClient; import com.loopj.android.http.RequestParams; import com.loopj.android.http.ResponseHandlerInterface; public class WebReq { public static AsyncHttpClient client; static{ //create object of loopj client //443 will save you from ssl exception client = new AsyncHttpClient(true,80,443); } public static void get(Context context, String url, RequestParams params, ResponseHandlerInterface responseHandler) { client.get(context, getAbsoluteUrl(url), params, responseHandler); } //concatenation of base url and file name private static String getAbsoluteUrl(String relativeUrl) { Log.d("response URL: ",GlobalClass.getInstance().BASE_URL + relativeUrl+" "); return GlobalClass.getInstance().BASE_URL + relativeUrl; } public static void post(Context context, String url, RequestParams params, ResponseHandlerInterface responseHandler) { client.post(context, getAbsoluteUrl(url), params, responseHandler); } }
9. AndroidManifest.xml Intent Filter
Intent-Filter (intent-filter) is used to make the specific activities as launcher activity. By adding intent-filter inside SplashActvity this will become our launcher activity and will run at the start of the application.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.tutorialscache.loginsignup"> <uses-permission android:name="android.permission.INTERNET"/> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"></activity> <activity android:name=".SplashActivity" android:screenOrientation="portrait"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name=".LoginActivity" android:screenOrientation="portrait" /> <activity android:name=".SignupActivity" android:screenOrientation="portrait" /> <activity android:name=".HomeActivity" android:screenOrientation="portrait"></activity> </application> </manifest>
10. MainActivity.java
MainActivity class will be used as a parent activity to all other activities in the app so as a result, we will access to MainActivity methods.
package com.tutorialscache.loginsignup; import android.content.Context; import android.content.Intent; import android.content.SharedPreferences; import android.support.v4.app.NavUtils; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.MenuItem; import com.loopj.android.http.AsyncHttpClient; import java.util.regex.Matcher; import java.util.regex.Pattern; public class MainActivity extends AppCompatActivity { Context context; Intent intent; SharedPreferences sharedPreferences; String SHARED_PREF_NAME ="user_pref"; SharedPreferences.Editor sharedPrefEditor; protected String name,email,password; protected boolean isLoggedIn(){ return sharedPreferences.getBoolean("login",false); } protected void logout(){ sharedPrefEditor.putBoolean("login",false); sharedPrefEditor.apply(); sharedPrefEditor.commit(); } public static boolean isEmailValid(String email) { String expression = "^[\\w\\.-]+@([\\w\\-]+\\.)+[A-Z]{2,4}$"; Pattern pattern = Pattern.compile(expression, Pattern.CASE_INSENSITIVE); Matcher matcher = pattern.matcher(email); return matcher.matches(); } public void init() { sharedPreferences = getSharedPreferences(SHARED_PREF_NAME,MODE_PRIVATE); sharedPrefEditor = sharedPreferences.edit(); } @Override public boolean onOptionsItemSelected(MenuItem item) { switch (item.getItemId()) { case android.R.id.home: finish(); default: return super.onOptionsItemSelected(item); } } }
11. activity_home.xml
Add the following code in its activity_home.xml file.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.tutorialscache.loginsignup.HomeActivity"> <TextView android:id="@+id/nameTv" android:text="Name" android:textSize="22dp" android:textStyle="bold" android:gravity="center_horizontal" android:layout_centerVertical="true" android:layout_width="match_parent" android:layout_height="wrap_content" /> <TextView android:id="@+id/emailTv" android:layout_below="@id/nameTv" android:text="e@e.com" android:textSize="22dp" android:gravity="center_horizontal" android:layout_centerVertical="true" android:layout_width="match_parent" android:layout_height="wrap_content" /> <Button android:id="@+id/logoutBtn" android:text="Logout" android:textAllCaps="false" android:background="#fa0e3d" android:textColor="#FFFFFF" android:layout_marginTop="40dp" android:layout_below="@id/emailTv" android:layout_centerHorizontal="true" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </RelativeLayout>
12. HomeActivity.java
package com.tutorialscache.loginsignup; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView; public class HomeActivity extends MainActivity { TextView nameTv; TextView emailTv; Button logoutbtn; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); getSupportActionBar().setTitle("Home"); context = this; init(); setContentView(R.layout.activity_home); //link views getViews(); } private void getViews() { nameTv = findViewById(R.id.nameTv); nameTv.setText(sharedPreferences.getString("name","")); emailTv = findViewById(R.id.emailTv); emailTv.setText(sharedPreferences.getString("email","")); logoutbtn = findViewById(R.id.logoutBtn); //make logout logoutbtn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { // Redirect back to login page logout(); intent = new Intent(context,LoginActivity.class); //remove all previous stack activities intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP); startActivity(intent); finish(); } }); } }
13. activity_splash.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.tutorialscache.loginsignup.SplashActivity"> <ProgressBar android:id="@+id/mPb" android:layout_centerHorizontal="true" android:layout_centerVertical="true" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <TextView android:text="@string/app_name" android:gravity="center_horizontal" android:layout_below="@+id/mPb" android:textSize="22dp" android:layout_width="match_parent" android:layout_height="wrap_content" /> </RelativeLayout>
14. SplashActivity.java
package com.tutorialscache.loginsignup; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import java.util.Timer; import java.util.TimerTask; public class SplashActivity extends MainActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); init(); setContentView(R.layout.activity_splash); // 5 seconds pause on splash page Timer timer = new Timer(); timer.schedule(new TimerTask() { @Override public void run() { if(isLoggedIn()){ //Redirect to home page intent = new Intent(context,HomeActivity.class); startActivity(intent); finish(); }else{ //Redirect to Login Page intent = new Intent(context,LoginActivity.class); startActivity(intent); finish(); } } },5000); } private void init() { context = this; sharedPreferences = context.getSharedPreferences(SHARED_PREF_NAME,MODE_PRIVATE); } }
15. activity_login.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.tutorialscache.loginsignup.LoginActivity"> <TextView android:id="@+id/titleTv" android:layout_centerHorizontal="true" android:layout_marginTop="120dp" android:text="Login" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <EditText android:id="@+id/emailEt" android:layout_below="@id/titleTv" android:layout_centerHorizontal="true" android:ems="11" android:hint="Email" android:inputType="textEmailAddress" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <EditText android:id="@+id/passwordEt" android:layout_below="@id/emailEt" android:layout_centerHorizontal="true" android:ems="11" android:hint="Password" android:inputType="number" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <Button android:id="@+id/loginBtn" android:text="Login" android:layout_marginTop="15dp" android:layout_centerHorizontal="true" android:background="#fa0e3d" android:textColor="#FFFFFF" android:layout_below="@id/passwordEt" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <TextView android:id="@+id/signupNowTv" android:gravity="right" android:textColor="#fa0e5d" android:padding="5dp" android:layout_below="@id/loginBtn" android:layout_marginTop="20dp" android:text="Don't have an acccount ? Signup Now ?" android:layout_width="match_parent" android:layout_height="wrap_content" /> </RelativeLayout>
14. LoginActivity.java
package com.tutorialscache.loginsignup; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; import android.widget.Toast; import com.loopj.android.http.AsyncHttpClient; import com.loopj.android.http.AsyncHttpResponseHandler; import com.loopj.android.http.JsonHttpResponseHandler; import com.loopj.android.http.RequestParams; import com.loopj.android.http.ResponseHandlerInterface; import org.json.JSONArray; import org.json.JSONException; import org.json.JSONObject; import cz.msebera.android.httpclient.Header; public class LoginActivity extends MainActivity { EditText emailEt,passwordEt; Button loginBtn; TextView signupNowTv; String email,password; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); context=this; setContentView(R.layout.activity_login); init(); getViews(); } private void getViews() { emailEt = findViewById(R.id.emailEt); signupNowTv = findViewById(R.id.signupNowTv); passwordEt = findViewById(R.id.passwordEt); loginBtn = findViewById(R.id.loginBtn); loginBtn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { loginValidation(); } }); signupNowTv.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { intent = new Intent(context,SignupActivity.class); startActivity(intent); } }); } private void loginValidation() { email = emailEt.getText().toString(); password = passwordEt.getText().toString(); if (email.length()==0){ Toast.makeText(context,"Invalid Email Address",Toast.LENGTH_SHORT).show(); return; } if (isEmailValid(email)==false){ Toast.makeText(context,"Invalid Email Address",Toast.LENGTH_SHORT).show(); return; } if (password.length()<5){ Toast.makeText(context,"Minimum password length should be 5 characters.",Toast.LENGTH_SHORT).show(); return; } //all inputs are validated now perform login request RequestParams params = new RequestParams(); params.add("type","login"); params.add("email",email); params.add("password",password); WebReq.get(context, "api.php", params, new LoginActivity.ResponseHandler()); } private void init() { context =this; sharedPreferences = getSharedPreferences(SHARED_PREF_NAME,MODE_PRIVATE); sharedPrefEditor = sharedPreferences.edit(); } private class ResponseHandler extends JsonHttpResponseHandler { @Override public void onStart() { super.onStart(); } @Override public void onSuccess(int statusCode, Header[] headers, JSONObject response) { super.onSuccess(statusCode, headers, response); Log.d("response ",response.toString()+" "); try { if (response.getBoolean("error")){ // failed to login Toast.makeText(context,response.getString("message"),Toast.LENGTH_SHORT).show(); }else{ // successfully logged in JSONObject user = response.getJSONObject("user"); //save login values sharedPrefEditor.putBoolean("login",true); sharedPrefEditor.putString("id",user.getString("id")); sharedPrefEditor.putString("name",user.getString("name")); sharedPrefEditor.putString("email",user.getString("email")); sharedPrefEditor.apply(); sharedPrefEditor.commit(); //Move to home activity intent = new Intent(context,HomeActivity.class); startActivity(intent); finish(); } } catch (JSONException e) { e.printStackTrace(); } } @Override public void onFailure(int statusCode, Header[] headers, String responseString, Throwable throwable) { super.onFailure(statusCode, headers, responseString, throwable); } @Override public void onFinish() { super.onFinish(); } } }
15. activity_signup.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@color/colorPrimary" tools:context="com.tutorialscache.loginsignup.LoginActivity"> <TextView android:id="@+id/titleTv" android:layout_centerHorizontal="true" android:layout_marginTop="120dp" android:text="Signup" android:textSize="20dp" android:textColor="@color/white" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <EditText android:id="@+id/nameEt" android:layout_below="@id/titleTv" android:layout_centerHorizontal="true" android:ems="11" android:background="@color/white" android:padding="8dp" android:layout_marginTop="10dp" android:layout_marginBottom="10dp" android:hint="Name" android:inputType="text" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <EditText android:id="@+id/emailEt" android:layout_below="@id/nameEt" android:layout_centerHorizontal="true" android:ems="11" android:background="@color/white" android:padding="8dp" android:hint="Email" android:layout_marginBottom="10dp" android:inputType="textEmailAddress" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <EditText android:id="@+id/passwordEt" android:layout_below="@id/emailEt" android:layout_centerHorizontal="true" android:ems="11" android:background="@color/white" android:padding="8dp" android:hint="Password" android:inputType="numberPassword" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <Button android:id="@+id/SignupBtn" android:text="Signup" android:textAllCaps="false" android:layout_marginTop="15dp" android:layout_centerHorizontal="true" android:background="@color/white" android:textColor="@color/colorPrimary" android:layout_below="@id/passwordEt" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <TextView android:id="@+id/LoginNowTv" android:gravity="right" android:textColor="@color/white" android:padding="5dp" android:layout_below="@id/SignupBtn" android:layout_marginTop="20dp" android:text="Already have an acccount ? Login ?" android:layout_width="match_parent" android:layout_height="wrap_content" /> </RelativeLayout>
16. SignupActivity.java
package com.tutorialscache.loginsignup; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; import android.widget.Toast; import com.loopj.android.http.JsonHttpResponseHandler; import com.loopj.android.http.RequestParams; import org.json.JSONException; import org.json.JSONObject; import cz.msebera.android.httpclient.Header; public class SignupActivity extends MainActivity { EditText nameEt,emailEt,passwordEt; Button signupBtn; TextView LoginNowTv; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); getSupportActionBar().setTitle("Registration"); getSupportActionBar().setDisplayHomeAsUpEnabled(true); context = this; init(); setContentView(R.layout.activity_signup); getViews(); } public void getViews() { nameEt = findViewById(R.id.nameEt); emailEt = findViewById(R.id.emailEt); passwordEt = findViewById(R.id.passwordEt); signupBtn = findViewById(R.id.SignupBtn); LoginNowTv = findViewById(R.id.LoginNowTv); signupBtn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { signupValidation(); } }); LoginNowTv.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { finish(); } }); } private void signupValidation() { name = nameEt.getText().toString(); email = emailEt.getText().toString(); password = passwordEt.getText().toString(); if (name.length()<3){ Toast.makeText(context,"Name at least 3 characters.",Toast.LENGTH_SHORT).show(); return; } if (email.length()==0){ Toast.makeText(context,"Invalid Email Address",Toast.LENGTH_SHORT).show(); return; } if (isEmailValid(email)==false){ Toast.makeText(context,"Invalid Email Address",Toast.LENGTH_SHORT).show(); return; } if (password.length()<5){ Toast.makeText(context,"Minimum password length should be 5 characters.",Toast.LENGTH_SHORT).show(); return; } //all inputs are validated now perform login request RequestParams params = new RequestParams(); params.add("type","signup"); params.add("name",name); params.add("email",email); params.add("password",password); WebReq.post(context, "api.php", params, new SignupActivity.ResponseHandler()); } private class ResponseHandler extends JsonHttpResponseHandler { @Override public void onStart() { super.onStart(); } @Override public void onSuccess(int statusCode, Header[] headers, JSONObject response) { super.onSuccess(statusCode, headers, response); Log.d("response ",response.toString()+" "); try { if (response.getBoolean("error")){ // failed to login Toast.makeText(context,response.getString("message"),Toast.LENGTH_SHORT).show(); }else{ // successfully logged in JSONObject user = response.getJSONObject("user"); //save login values sharedPrefEditor.putBoolean("login",true); sharedPrefEditor.putString("id",user.getString("id")); sharedPrefEditor.putString("name",user.getString("name")); sharedPrefEditor.putString("email",user.getString("email")); sharedPrefEditor.apply(); sharedPrefEditor.commit(); //Move to home activity intent = new Intent(context,HomeActivity.class); intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP); startActivity(intent); finish(); } } catch (JSONException e) { e.printStackTrace(); } } @Override public void onFailure(int statusCode, Header[] headers, String responseString, Throwable throwable) { super.onFailure(statusCode, headers, responseString, throwable); } @Override public void onFinish() { super.onFinish(); } } }
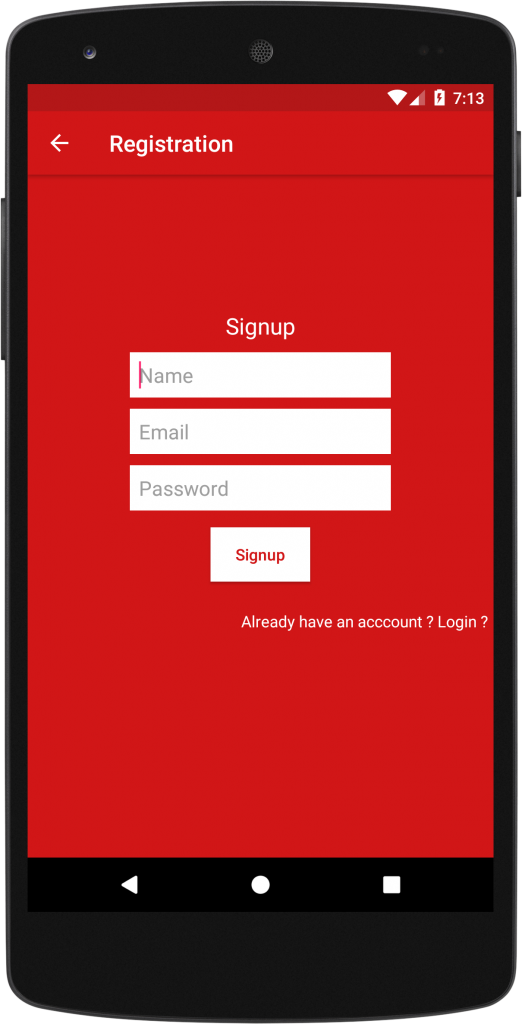
Comments are closed.